Node:Yes-or-No Questions, Previous:Formatting Numbers, Up:Locales
In the GNU C library, stdin, stdout, and stderr are normal variables which you can set just like any others. For example, to redirect the standard output to a file, you could do: fclose (stdout); stdout = fopen ('standard-output-file', 'w'). It was discovered that the GNU C Library incorrectly handled certain signal trampolines on PowerPC. A remote attacker could use this issue to cause the GNU C Library to crash, resulting in a denial of service, or possibly execute arbitrary code. (CVE-2020-1751) It was discovered that the GNU C Library incorrectly handled tilde expansion.
How To Install Gnu C Library On Ubuntu
Yes-or-No Questions
Some non GUI programs ask a yes-or-no question. If the messages (especially the questions) are translated into foreign languages, be sure that you localize the answers too. It would be very bad habit to ask a question in one language and request the answer in another, often English.
The GNU C library contains rpmatch
to give applications easy access to the corresponding locale definitions.
int rpmatch (const char *response) | Function |
Gnu C Library For Windows
The function rpmatch checks the string in response whether or not it is a correct yes-or-no answer and if yes, which one. The check uses the YESEXPR and NOEXPR data in the LC_MESSAGES category of the currently selected locale. The return value is as follows:
This function is not standardized but available beside in GNU libc at least also in the IBM AIX library. |
This function would normally be used like this:
Note that the loop continues until an read error is detected or until a definitive (positive or negative) answer is read.
Gnu C Library Online
Table of Contents
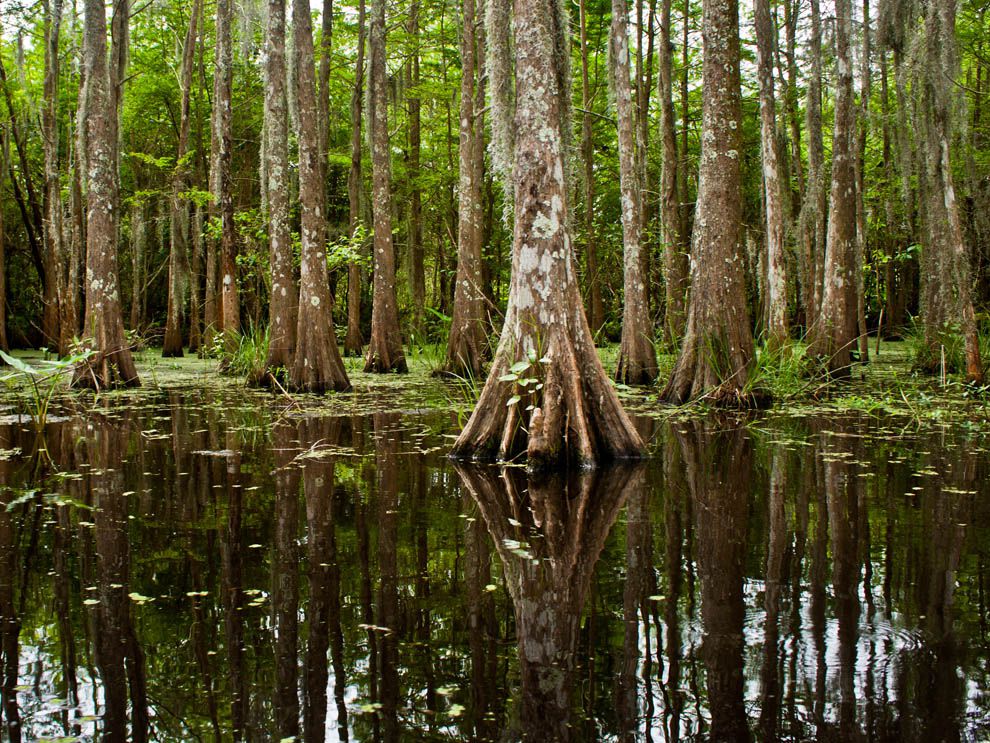
- I. Introduction
- 1. Status
- Implementation Status
- C++ 1998/2003
- Implementation Status
- Implementation Specific Behavior
- C++ 2011
- Implementation Specific Behavior
- C++ 2014
- C++ 2017
- Implementation Specific Behavior
- C++ 202a
- Implementation Specific Behavior
- C++ TR1
- Implementation Specific Behavior
- C++ TR 24733
- C++ IS 29124
- Implementation Specific Behavior
- License
- The Code: GPL
- The Documentation: GPL, FDL
- Bugs
- Implementation Bugs
- Standard Bugs
- 2. Setup
- Prerequisites
- Configure
- Make
- 3. Using
- Command Options
- Headers
- Header Files
- Mixing Headers
- The C Headers and
namespace std
- Precompiled Headers
- Macros
- Dual ABI
- Troubleshooting
- Namespaces
- Available Namespaces
- namespace std
- Using Namespace Composition
- Linking
- Almost Nothing
- Finding Dynamic or Shared Libraries
- Experimental Library Extensions
- Concurrency
- Prerequisites
- Thread Safety
- Atomics
- IO
- Structure
- Defaults
- Future
- Alternatives
- Containers
- Exceptions
- Exception Safety
- Exception Neutrality
- Doing without
- Compatibility
- With
C
- With
POSIX
thread cancellation
- With
- Debugging Support
- Using g++
- Debug Versions of Library Binary Files
- Memory Leak Hunting
- Non-memory leaks in Pool and MT allocators
- Data Race Hunting
- Using gdb
- Tracking uncaught exceptions
- Debug Mode
- Compile Time Checking
- Profile-based Performance Analysis
- II. Standard Contents
- 4. Support
- Types
- Fundamental Types
- Numeric Properties
- NULL
- Dynamic Memory
- Termination
- Termination Handlers
- Verbose Terminate Handler
- 5. Diagnostics
- Exceptions
- API Reference
- Adding Data to
exception
- Use of errno by the library
- Concept Checking
- 6. Utilities
- Functors
- Pairs
- Memory
- Allocators
- Requirements
- Design Issues
- Implementation
- Interface Design
- Selecting Default Allocation Policy
- Disabling Memory Caching
- Using a Specific Allocator
- Custom Allocators
- Extension Allocators
- auto_ptr
- Limitations
- Use in Containers
- shared_ptr
- Requirements
- Design Issues
- Implementation
- Class Hierarchy
- Thread Safety
- Selecting Lock Policy
- Related functions and classes
- Use
- Examples
- Unresolved Issues
- Acknowledgments
- Traits
- 7. Strings
- String Classes
- Simple Transformations
- Case Sensitivity
- Arbitrary Character Types
- Tokenizing
- Shrink to Fit
- CString (MFC)
- 8. Localization
- Locales
- locale
- Requirements
- Design
- Implementation
- Interacting with 'C' locales
- Future
- Facets
- ctype
- Implementation
- Specializations
- Future
- codecvt
- Requirements
- Design
- wchar_t Size
- Support for Unicode
- Other Issues
- Implementation
- Use
- Future
- messages
- Requirements
- Design
- Implementation
- Models
- The GNU Model
- Use
- Future
- 9. Containers
- Sequences
- list
- list::size() is O(n)
- Associative
- Insertion Hints
- bitset
- Size Variable
- Type String
- Unordered Associative
- Insertion Hints
- Hash Code
- Hash Code Caching Policy
- Interacting with C
- Containers vs. Arrays
- 10. Iterators
- Predefined
- Iterators vs. Pointers
- One Past the End
- 11. Algorithms
- Mutating
swap
- Specializations
- 12. Numerics
- Complex
- complex Processing
- Generalized Operations
- Interacting with C
- Numerics vs. Arrays
- C99
- 13. Input and Output
- Iostream Objects
- Stream Buffers
- Derived streambuf Classes
- Buffering
- Memory Based Streams
- Compatibility With strstream
- File Based Streams
- Copying a File
- Binary Input and Output
- Interacting with C
- Using FILE* and file descriptors
- Performance
- 14. Atomics
- API Reference
- 15. Concurrency
- API Reference
- III. Extensions
- 16. Compile Time Checks
- 17. Debug Mode
- Intro
- Semantics
- Using
- Using the Debug Mode
- Using a Specific Debug Container
- Design
- Goals
- Methods
- The Wrapper Model
- Safe Iterators
- Safe Sequences (Containers)
- Precondition Checking
- Release- and debug-mode coexistence
- Compile-time coexistence of release- and debug-mode components
- Link- and run-time coexistence of release- and debug-mode components
- Alternatives for Coexistence
- Other Implementations
- 18. Parallel Mode
- Intro
- Semantics
- Using
- Prerequisite Compiler Flags
- Using Parallel Mode
- Using Specific Parallel Components
- Design
- Interface Basics
- Configuration and Tuning
- Setting up the OpenMP Environment
- Compile Time Switches
- Run Time Settings and Defaults
- Implementation Namespaces
- Testing
- Bibliography
- 19. Profile Mode
- Intro
- Using the Profile Mode
- Tuning the Profile Mode
- Design
- Wrapper Model
- Instrumentation
- Run Time Behavior
- Analysis and Diagnostics
- Cost Model
- Reports
- Testing
- Extensions for Custom Containers
- Empirical Cost Model
- Implementation Issues
- Stack Traces
- Symbolization of Instruction Addresses
- Concurrency
- Using the Standard Library in the Instrumentation Implementation
- Malloc Hooks
- Construction and Destruction of Global Objects
- Developer Information
- Big Picture
- How To Add A Diagnostic
- Diagnostics
- Diagnostic Template
- Containers
- Hashtable Too Small
- Hashtable Too Large
- Inefficient Hash
- Vector Too Small
- Vector Too Large
- Vector to Hashtable
- Hashtable to Vector
- Vector to List
- List to Vector
- List to Forward List (Slist)
- Ordered to Unordered Associative Container
- Algorithms
- Sort Algorithm Performance
- Data Locality
- Need Software Prefetch
- Linked Structure Locality
- Multithreaded Data Access
- Data Dependence Violations at Container Level
- False Sharing
- Statistics
- Bibliography
- 20. The mt_allocator
- Intro
- Design Issues
- Overview
- Implementation
- Tunable Parameters
- Initialization
- Deallocation Notes
- Single Thread Example
- Multiple Thread Example
- 21. The bitmap_allocator
- Design
- Implementation
- Free List Store
- Super Block
- Super Block Data Layout
- Maximum Wasted Percentage
allocate
deallocate
- Questions
- 1
- 2
- 3
- Locality
- Overhead and Grow Policy
- 22. Policy-Based Data Structures
- Intro
- Performance Issues
- Associative
- Priority Que
- Goals
- Associative
- Policy Choices
- Underlying Data Structures
- Iterators
- Functional
- Priority Queues
- Policy Choices
- Underlying Data Structures
- Binary Heaps
- Using
- Prerequisites
- Organization
- Tutorial
- Basic Use
- Configuring via Template Parameters
- Querying Container Attributes
- Point and Range Iteration
- Examples
- Intermediate Use
- Querying with
container_traits
- By Container Method
- Hash-Based
- Branch-Based
- Priority Queues
- Design
- Concepts
- Null Policy Classes
- Map and Set Semantics
- Distinguishing Between Maps and Sets
- Alternatives to
std::multiset
andstd::multimap
- Iterator Semantics
- Point and Range Iterators
- Distinguishing Point and Range Iterators
- Invalidation Guarantees
- Genericity
- Tag
- Traits
- By Container
- hash
- Interface
- Details
- tree
- Interface
- Details
- Trie
- Interface
- Details
- List
- Interface
- Details
- Priority Queue
- Interface
- Details
- Testing
- Regression
- Performance
- Hash-Based
- Text
find
- Integer
find
- Integer Subscript
find
- Integer Subscript
insert
- Integer
find
with Skewed-Distribution - Erase Memory Use
- Text
- Branch-Based
- Text
insert
- Text
find
- Text
find
with Locality-of-Reference split
andjoin
- Order-Statistics
- Text
- Multimap
- Text
find
with Small Secondary-to-Primary Key Ratios - Text
find
with Large Secondary-to-Primary Key Ratios - Text
insert
with Small Secondary-to-Primary Key Ratios - Text
insert
with Small Secondary-to-Primary Key Ratios - Text
insert
with Small Secondary-to-Primary Key Ratios Memory Use - Text
insert
with Small Secondary-to-Primary Key Ratios Memory Use
- Text
- Priority Queue
- Text
push
- Text
push
andpop
- Integer
push
- Integer
push
- Text
pop
Memory Use - Text
join
- Text
modify
Up - Text
modify
Down
- Text
- Observations
- Associative
- Priority_Queue
- Acknowledgments
- Bibliography
- 23. HP/SGI Extensions
- Backwards Compatibility
- Deprecated
- 24. Utilities
- 25. Algorithms
- 26. Numerics
- 27. Iterators
- 28. Input and Output
- Derived filebufs
- 29. Demangling
- 30. Concurrency
- Design
- Interface to Locks and Mutexes
- Interface to Atomic Functions
- Implementation
- Using Built-in Atomic Functions
- Thread Abstraction
- Use
- IV. Appendices
- A. Contributing
- Contributor Checklist
- Reading
- Assignment
- Getting Sources
- Submitting Patches
- Directory Layout and Source Conventions
- Coding Style
- Bad Identifiers
- By Example
- Design Notes
- B. Porting and Maintenance
- Configure and Build Hacking
- Prerequisites
- Overview
- General Process
- What Comes from Where
- Configure
- Storing Information in non-AC files (like configure.host)
- Coding and Commenting Conventions
- The acinclude.m4 layout
GLIBCXX_ENABLE
, the--enable
maker- Shared Library Versioning
- Make
- Writing and Generating Documentation
- Introduction
- Generating Documentation
- Doxygen
- Prerequisites
- Generating the Doxygen Files
- Debugging Generation
- Markup
- Docbook
- Prerequisites
- Generating the DocBook Files
- Debugging Generation
- Editing and Validation
- File Organization and Basics
- Markup By Example
- Porting to New Hardware or Operating Systems
- Operating System
- CPU
- Character Types
- Thread Safety
- Numeric Limits
- Libtool
- Testing
- Test Organization
- Directory Layout
- Naming Conventions
- Running the Testsuite
- Basic
- Variations
- Permutations
- Writing a new test case
- Examples of Test Directives
- Directives Specific to Libstdc++ Tests
- Test Harness and Utilities
- DejaGnu Harness Details
- Utilities
- Special Topics
- Qualifying Exception Safety Guarantees
- Overview
- Existing tests
- C++11 Requirements Test Sequence Descriptions
- ABI Policy and Guidelines
- The C++ Interface
- Versioning
- Goals
- History
- Prerequisites
- Configuring
- Checking Active
- Allowed Changes
- Prohibited Changes
- Implementation
- Testing
- Single ABI Testing
- Multiple ABI Testing
- Outstanding Issues
- API Evolution and Deprecation History
3.0
3.1
3.2
3.3
3.4
4.0
4.1
4.2
4.3
4.4
4.5
4.6
4.7
4.8
4.9
5
5.3
6
7
7.2
7.3
8
9
- Backwards Compatibility
- First
- No
ios_base
- No
cout
in<ostream.h>
, nocin
in<istream.h>
- No
- Second
- Namespace
std::
not supported - Illegal iterator usage
isspace
from<cctype>
is a macro- No
vector::at
,deque::at
,string::at
- No
std::char_traits<char>::eof
- No
string::clear
- Removal of
ostream::form
andistream::scan
extensions - No
basic_stringbuf
,basic_stringstream
- Little or no wide character support
- No templatized iostreams
- Thread safety issues
- Namespace
- Third
- Pre-ISO headers removed
- Extension headers hash_map, hash_set moved to ext or backwards
- No
ios::nocreate/ios::noreplace
. - No
stream::attach(int fd)
- Support for C++98 dialect.
- Support for C++TR1 dialect.
- Support for C++11 dialect.
Container::iterator_type
is not necessarilyContainer::value_type*
- C. Free Software Needs Free Documentation
- D. GNU General Public License version 3
- E. GNU Free Documentation License